API and Pokemon
Image by Jordi, Made with AI
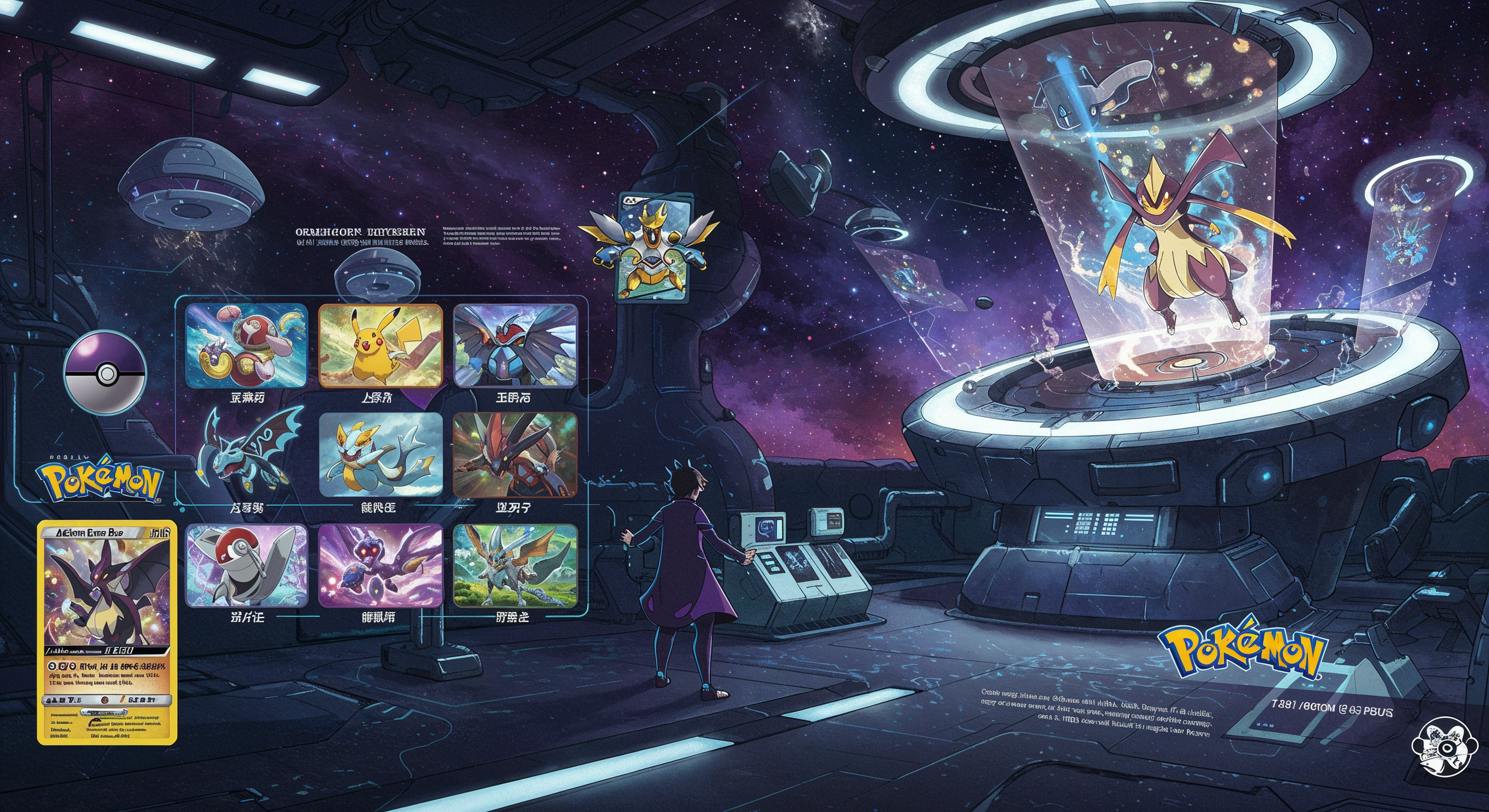
Pokémon API Astro App Documentation
Overview
My goal was to gain knowledge about Astro and APIs through the process of creating this project. Additionally, I will document all the APIs I use for my project and comprehend how to use the documentation.
This application displays a list of Pokémon fetched from the PokéAPI. By default, it retrieves the first 151 Pokémon. It uses Astro’s static-site generation features to paginate the list and generate detail pages for each Pokémon. Users can also mark certain Pokémon as “favorites,” stored locally in the browser’s localStorage
.
Here is the website example: Pokémon App
All the code is in my GitHub: GitHub Repository
Technology Stack
-
Astro
Static Site Generation & page routing -
TypeScript/JavaScript
Client-side scripts for favorites and page transitions -
CSS
Tailwind classes for styling -
PokéAPI
https://pokeapi.co -
Postman (optional)
For testing the external API
Project File Structure
A simplified look at the main files involved:
src/
├─ pages/
│ ├─ index.astro // Redirects to /1
│ ├─ [page].astro // Paginated listing of Pokémon
│ └─ pokemon/
│ └─ [id].astro // Detail page for a single Pokémon
│
├─ components/
│ └─ pokemons/
│ └─ PokemonCard.astro // Renders a Pokémon card
│
├─ layouts/
│ └─ MainLayout.astro // Base HTML layout (header, body, etc.)
│
├─ interfaces/
│ ├─ pokemon-list.response.ts // Type definitions for API responses
│ └─ favorite-pokemon.ts // Type definition for favorites
│
└─ styles/
└─ global.css // Global styling (Tailwind, etc.)
Fetching and Displaying Pokémon
The PokéAPI Request
-
Endpoint:
https://pokeapi.co/api/v2/pokemon?limit=151
-
Purpose:
Retrieves a JSON list of the first 151 Pokémon (names and URLs). -
Example Response (truncated):
{
"count": 1281,
"next": "...",
"previous": null,
"results": [
{
"name": "bulbasaur",
"url": "https://pokeapi.co/api/v2/pokemon/1/"
},
{
"name": "ivysaur",
"url": "https://pokeapi.co/api/v2/pokemon/2/"
}
]
}
How to Get Data from PokéAPI
We want to use the Pokémon sound and the name, and PokéAPI can provide both. Here are the steps to do it:
- Go to PokéAPI Documentation and select “Pokemon” on the left.
- Copy the
GET
request:https://pokeapi.co/api/v2/pokemon/{id or name}/
. - There are 1164 Pokémon as of 2025. We can test the API in Postman to see if the link works. To do that, follow these steps:
- Open Postman and create a new request.
- Paste the link
https://pokeapi.co/api/v2/pokemon/{id or name}/
and replace{id or name}
with a Pokémon ID (e.g.,150
for Mewtwo). - Choose GET, then click Send to see the Pokémon’s details.
Pokémon Sound Example
Here you can find the sound of the Pokémon, which can be used on the website:
Pokémon Sound (Mewtwo)
Testing with Postman
To retrieve all 151 Pokémon:
- Open Postman.
- Make a GET request to:
https://pokeapi.co/api/v2/pokemon?limit=151
- Confirm you receive a 200 OK response with a JSON body containing 151 Pokémon.
- (Optional) Adjust query parameters or explore additional data.
Pages and Components
index.astro
- Role: Automatically redirects the user to the paginated listing (e.g.,
/1
). - Key Points:
export function getStaticProps() {
return {
redirect: '/1',
};
}
[page].astro
(Paginated Listing)
- Purpose: Displays 12 Pokémon per page, using Astro’s built-in pagination.
- How it Works:
getStaticPaths
fetches?limit=151
.- Astro’s
paginate
function splits the list into pages of size 12. - Each page automatically gets a route:
/1
,/2
,/3
, etc. - Uses
<PokemonCard />
to show each Pokémon’s image and name.
- Navigation:
- Links for Previous and Next pages.
- The current page is shown at the bottom.
[id].astro
(Detail Page)
- Purpose: Shows details for a single Pokémon by ID (1–151).
- How it Works:
getStaticPaths
fetches the same 151 Pokémon, mapping each to an ID extracted from the URL.- The page URL is
/pokemon/[id]
, e.g.,/pokemon/25
for Pikachu. - Renders:
- Official artwork from GitHub (based on the ID).
- A playable audio cry (from a separate GitHub repository).
- A favorite heart icon toggling
localStorage
data.
PokemonCard.astro
- Purpose: Card component to display a single Pokémon (image + name).
- Logic:
- Extracts the Pokémon ID from the URL.
- Builds a detail-page link like:
so the user can return to the correct listing page./pokemon/${id}?page=${currentPage ?? 1}
MainLayout.astro
- Purpose: Wraps all pages with a consistent HTML structure (head, meta tags, nav bar).
- Key Points:
- Receives props like
title
,description
,image
for SEO. - Uses
<NavBar />
for top navigation. - Loads Tailwind classes from
global.css
.
- Receives props like
Running the Project Locally
You can run this project and test it by following these steps:
-
Clone the repository or copy all files into a local folder.
-
Install dependencies:
npm install
-
Run Astro dev server:
npm run dev
-
Open http://localhost:4321 in your browser.
-
You should see the Pokémon listing. Use Next and Previous to paginate.
-
API and Pokemon
-
Creating Peachjar’s Help Center
-
Astro: Lessons & Wins
-
Twitter Mention bot
-
Astro Test Blog
-
Hubspot-Modules
-
HubSpot-Module-RichText
Related:
Written by
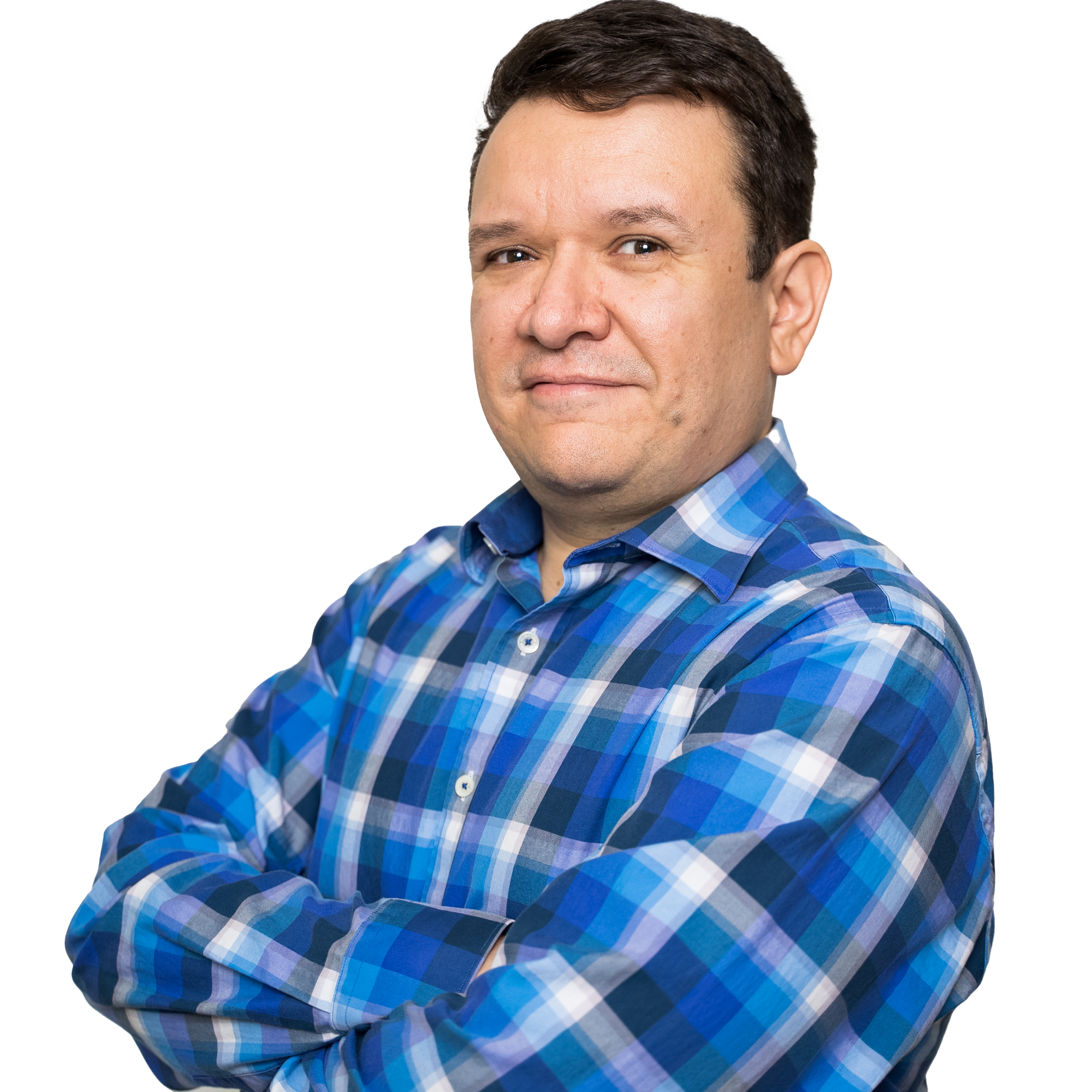
Jordi Comas
Senior Technical Writer
Industrial Designer